How to Truncate dangerouslySetInnerHTML in React
Author:
cmsgranit
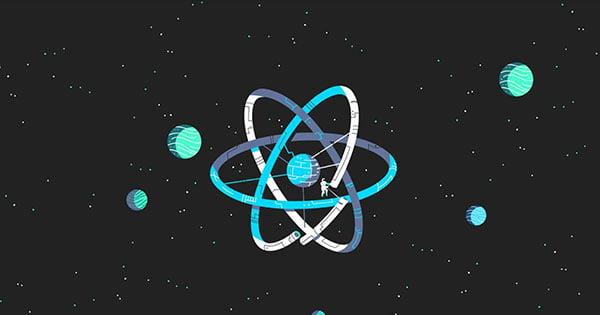
When working with React, you may encounter situations where you need to render HTML content as a string using dangerouslySetInnerHTML
. This can be useful for rendering user-generated content, but it also introduces potential security vulnerabilities if the content is not properly sanitized.
In this article, we’ll explore how to truncate dangerouslySetInnerHTML
in React using a function component.
Example Function Component
Here’s an example of a function component that takes in the HTML content and a maximum length, and renders the content truncated to the specified length:
function TruncatedHtml({ content, maxLength }) {
if (content.length <= maxLength) {
return <div dangerouslySetInnerHTML={{ __html: content }} />;
}
const truncatedContent = content.substring(0, maxLength) + '...';
return <div dangerouslySetInnerHTML={{ __html: truncatedContent }} />;
}
JavaScriptLet’s break down how this function component works.
First, the component checks whether the length of the HTML content is less than or equal to the specified maximum length. If it is, the component simply renders the content using dangerouslySetInnerHTML
.
If the length of the content is greater than the specified maximum length, the component truncates the content by using the substring
method to extract a substring of the first maxLength
characters, and then append an ellipsis at the end.
Finally, the component renders the truncated content using dangerouslySetInnerHTML
.
Usage Example
Here’s an example of how to use the TruncatedHtml
the component in your React application:
function App() {
const content = '<p>This is some HTML content that needs to be truncated.</p>';
const maxLength = 30;
return <TruncatedHtml content={content} maxLength={maxLength} />;
}
JavaScriptIn this example, we define a content
variable that contains some HTML content that needs to be truncated, and a maxLength
variable that specifies the maximum length of the truncated content. We then render the TruncatedHtml
component and pass in the content
and maxLength
props.
When the TruncatedHtml
component is rendered, it will truncate the content
to the specified maxLength
and render the truncated content using dangerouslySetInnerHTML
.
Conclusion
In this article, we’ve explored how to truncate dangerouslySetInnerHTML
in React using a function component. By truncating the HTML content before rendering it, we can reduce the risk of security vulnerabilities and improve the overall security of our React applications.
Keep in mind that truncating HTML content can result in incomplete tags or invalid HTML, which may cause rendering issues or unexpected behavior. Additionally, truncating the content may not address all potential security vulnerabilities that dangerouslySetInnerHTML
can introduce, so it’s generally recommended to use safer alternatives, such as React’s built-in JSX syntax or a third-party library that provides safer HTML rendering.
You might also enjoy
How to Add Author and Featured...
Enhancing the WordPress REST API: Adding Author and Featu...
How to Truncate dangerouslySet...
When working with React, you may encounter situations whe...
Building a Multilingual Next.j...
Polylang is a popular WordPress plugin that allows users ...
Comments (0)
No comments yet!
Speak up and share your thoughts! Your comments are valuable to us and help us improve.writeComment